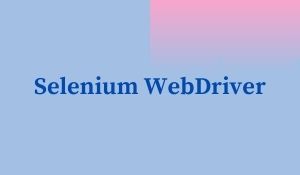
1. What are the significant changes in upgrades in various Selenium versions?
Selenium v1 included only three suites of tools: Selenium IDE, Selenium RC and Selenium Grid. Note that there was no WebDriver in Selenium v1. Selenium WebDriver was introduced in Selenium v2. With the onset of WebDriver, Selenium RC got deprecated and is not in use since. Older versions of RC are available in the market though, but support for RC is not available. Currently, Selenium v3 is in use, and it comprises IDE, WebDriver and Grid. Selenium 4 is actually the latest version.
IDE is used for recording and playback of tests, WebDriver is used for testing dynamic web applications via a programming interface and Grid is used for deploying tests in remote host machines.
2. Explain the different exceptions in Selenium WebDriver.
Exceptions in Selenium are similar to exceptions in other programming languages. The most common exceptions in Selenium are:
•TimeoutException: This exception is thrown when a command performing an operation does not complete in the stipulated time.
•NoSuchElementException: This exception is thrown when an element with given attributes is not found on the web page.
•ElementNotVisibleException: This exception is thrown when the element is present in DOM (Document Object Model), but not visible on the web page.
•StaleElementException: This exception is thrown when the element is either deleted or no longer attached to the DOM.
3. What is an exception test in Selenium?
An exception test is an exception that you expect will be thrown inside a test class. If you have written a test case in such a way that it should throw an exception, then you can use the @Test annotation and specify which exception you will be expecting by mentioning it in the parameters. Take a look at the example below:
@Test(expectedException = NoSuchElementException.class)
Do note the syntax, where the exception is suffixed with .class
4. Why and how will you use an Excel Sheet in your project?
The reason we use Excel sheets is because it can be used as a data source for tests. An excel sheet can also be used to store the data set while performing DataDriven Testing. These are the two main reasons for using Excel sheets.
When you use the excel sheet as data source, you can store the following:
•Application URL for all environments: You can specify the URL of the environment in which you want to do the testing like: development environment or testing environment or QA environment or staging environment or production/ pre-production environment.
•Username and password credentials of different environments: You can store the access credentials of the different applications/ environments in the excel sheet. You can store them in encoded format and whenever you want to use them, you can decode them instead of leaving it plain and unprotected.
•Test cases to be executed: You can list down the entire set of test cases in a column and in the next column, you can specify either Yes or No which indicates if you want that particular test case to be executed or ignored.
When you use the excel sheet for DataDriven Test, you can store the data for different iterations to be performed in the tests. For example while testing a web page, the different sets of input data that needs to be passed to the test box can be stored in the excel sheet.
5. What is POM (Page Object Model)?
Page Object Model is a design pattern for creating an Object Repository for web UI elements. Each web page in the application is required to have it’s own corresponding page class. The page class is thus responsible for finding the WebElements in that page and then performing operations on those WebElements.
6. What are the advantages of POM?
The advantages of using POM are:
•Allows us to separate operations and flows in the UI from Verification – improves code readability.
•Since the Object Repository is independent of Test Cases, multiple tests can use the same Object Repository.
•Reusability of code.
7. What are the different types of WAIT statements in Selenium WebDriver?
There are basically two types of wait statements: Implicit Wait and Explicit Wait.
Implicit wait instructs the WebDriver to wait for some time by polling the DOM. Once you have declared implicit wait, it will be available for the entire life of the WebDriver instance. By default, the value will be 0. If you set a longer default, then the behavior will poll the DOM on a periodic basis depending on the browser/ driver implementation.
Explicit wait instructs the execution to wait for some time until some condition is achieved. Some of those conditions to be attained are:
•elementToBeClickable
•elementToBeSelected
•presenceOfElementLocated
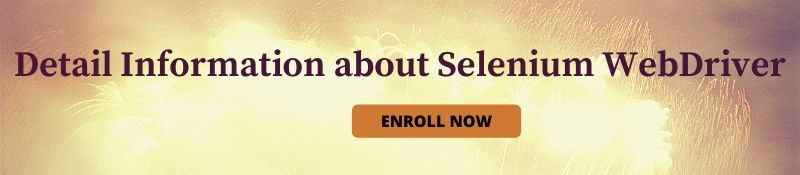
8. What are different types of frameworks?
The different types of frameworks are:
•Data Driven Framework: When the entire test data is generated from some external files like Excel, CSV, XML or some database table, then it is called Data Driven framework.
•Keyword Driven Framework: When only the instructions and operations are written in a different file like an Excel worksheet, it is called Keyword Driven framework.
•Hybrid Framework: A combination of both the Data Driven framework and the Keyword Driven framework is called Hybrid framework.
9. Which files can be used as a data source for different frameworks?
Some of the file types of the dataset can be: excel, xml, text, csv, etc.
10. What is Page Factory?
Page Factory gives an optimized way to implement Page Object Model. When we say it is optimized, it refers to the fact that the memory utilization is very good and also the implementation is done in an object oriented manner.
11.What is Selenese?
Selenese is the set of selenium commands which are used to test your web application.
You can even make use of:
•Actions: It is used for performing operations
•Assertions: This is Used as checkpoints
•Accessors: It is used for storing a value in a particular variable.
12. Can Selenium handle window pop-ups?
Selenium does not support handling pop-ups. Alert is used to display a warning message. It is a pop-up window that comes up on the screen.
A few methods using which this can be achieved:
•Void dismiss(): This method is called when the ‘Cancel’ button is clicked in the alert box.
•Void accept(): This method is called when you click on the ‘OK’ button of the alert.
•String getText(): This method is called to capture the alert message.
•Void sendKeys(String stringToSed): This is called when you want to send some data to the alert box.
13. What is a Robot class?
This Robot class provides control over the mouse and keyboard devices.
The methods include:
•KeyPress(): This method is called when you want to press any key.
•KeyRelease(): This method is used to release the pressed key on the keyboard.
•MouseMove(): This method is called when you want to move the mouse pointer in the X and Y coordinates.
•MousePress(): This is used to press the left button of the mouse.
•MouseMove(): This method helps in releasing the pressed button of the mouse.
14. How to handle multiple windows in Selenium?
A window handle is a unique identifier that holds the address of all the windows. This is basically a pointer to a window, which returns the string value.
•get.window handle(): This helps in getting the window handle of the current window.
•get.window handles(): It helps in getting the handles of all the windows opened.
•set: This helps to set the window handles which are in the form of a string.
•switch to: It helps in switching between the windows.
•action: This helps to perform certain actions on the windows.
15. What are Listeners in Selenium?
It is defined as an interface that modifies the behavior of the system. Listeners allow customization of reports and logs.
Listeners mainly comprise of two types, namely
•WebDriver listeners
•TestNG listeners
16. What are Assert and Verify commands?
•Assert: An assertion is used to compare the actual result of an application with the expected result.
•Verify: There won’t be any halt in the test execution even though the verify condition is true or false.
17. Can you navigate back and forth the webpage in Selenium?
Yes. You can navigate in the browser. A few methods using which you can achieve it are:
•driver.navigate.forward
•driver.manage.back
•driver.manage.navigate
•driver.navigate.to(“url”)
18. Explain how you can find broken links in a page using Selenium WebDriver?
This is a tricky question which the interviewer will present to you. He can provide a situation where there are 20 links in a web page, and we have to verify which of those 20 links are working and how many are not working (broken).
Since you need to verify the working of every link, the workaround is that, you need to send HTTP requests to all of the links on the web page and analyze the response. Whenever you use driver.get() method to navigate to a URL, it will respond with a status of 200 – OK. 200 – OK denotes that the link is working and it has been obtained. If any other status is obtained, then it is an indication that the link is broken.
We can do this. First, we have to use the anchor tags <a> to determine the different hyperlinks on the web page. For each <a> tag, we can use the attribute ‘href’ value to obtain the hyperlinks and then analyze the response received for each hyperlink when used in driver.get() method.
19. How to take screenshots in Selenium WebDriver?
Here, You can take a screenshot by using the TakeScreenshot function. By using getScreenshotAs() method you can save that screenshot.
For Example: File scrFile = ((TakeScreenshot)driver).getScreenshotAs(outputType.FILE);
20. Which technique should you consider using throughout the script “if there is neither frame id nor frame name”?
If neither frame name nor frame id is available, then we can use frame by index.
Let’s say that there are 3 frames in a web page and if none of them have a frame name and frame id, then we can still select those frames by using frame (zero-based) index attribute. Each frame will have an index number. The first frame would be at index “0”, the second at index “1” and the third at index “2”. Once the frame has been selected, all subsequent calls on the WebDriver interface will be made to that frame.
driver.switchTo().frame(int arg0);
21. What is the significance of testng.xml?
Since Selenium does not support report generation and test case management, we use the TestNG framework with Selenium. TestNG is much more advanced than JUnit, and it makes implementing annotations easy. That is the reason TestNG framework is used with Selenium WebDriver.
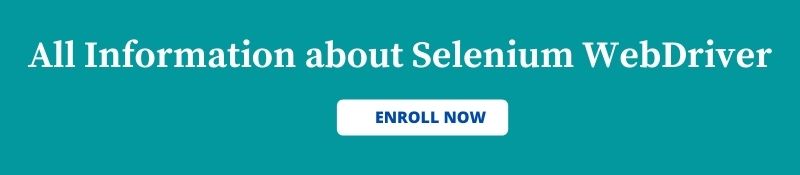
22. Where to define the test suites and grouping of test classes in TestNG?
It is by taking instructions from the testng.xml file. We cannot define a test suite in testing source code, instead it is represented in an XML file, because suite is the feature of execution. The test suite that I am talking about is basically a collection of test cases.
So for executing the test cases in a suite, i.e a group of test cases, you have to create a testng.xml file which contains the name of all the classes and methods that you want to execute as a part of that execution flow.
Other advantages of using testng.xml file are:
•It allows execution of multiple test cases from multiple classes
•It allows parallel execution
•It allows execution of test cases in groups, where a single test can belong to multiple groups.
23. Explain DataProviders in TestNG using an example. Can I call a single data provider method for multiple functions and classes?
•DataProvider is a TestNG feature, which enables us to write DataDriven tests. When we say, it supports DataDriven testing, then it becomes obvious that the same test method can run multiple times with different data-sets. DataProvider is in fact another way of passing parameters to the test method.
•@DataProvider marks a method as supplying data for a test method. The annotated method must return an Object[] where each Object[] can be assigned to the parameter list of the test method.
•To use the DataProvider feature in your tests, you have to declare a method annotated by @DataProvider and then use the said method in the test method using the ‘dataProvider‘ attribute in the Test annotation.
•As far as the second part of the question is concerned, Yes, the same DataProvider can be used in multiple functions and classes by declaring DataProvider in separate class and then reusing it in multiple classes.
24. How to skip a method or a code block in TestNG?
If you want to skip a particular test method, then you can set the ‘enabled’ parameter in test annotation to false.
@Test(enabled = false)
By default, the value of ‘enabled’ parameter will be true. Hence it is not necessary to define the annotation as true while defining it.
25. What is a soft assertion in Selenium? How can you mark a test case as failed by using soft assertion?
Soft Assertions are customized error handlers provided by TestNG. Soft Assertions do not throw exceptions when assertion fails, and they simply continue to the next test step. They are commonly used when we want to perform multiple assertions.
To mark a test as failed with soft assertions, call assertAll() method at the end of the test.
26. How to build the object repository?
An object repository is a common storage location for all objects. In the Selenium WebDriver context, objects would typically be the locators used to uniquely identify web elements.
27. How do you achieve synchronization in WebDriver?
It is a mechanism which involves more than one component to work parallel with each other.
Synchronization can be classified into two categories:
•Unconditional: In this we just specify timeout value only. We will make the tool to wait until a certain amount of time and then proceed further.
•Conditional: It specifies a condition along with timeout value, so that tool waits to check for the condition and then come out if nothing happens.
28. What are different types of TestNG Listeners in Selenium?
•IAnnotationTransformer
•IAnnotationTransformer2
•IConfigurable
•IConfigurationListener
•IExecutionListener
•IHookable
•IInvokedMethodListener
•IInvokedMethodListener2
•IMethodInterceptor
•IReporter
•ISuiteListener
•ITestListener
29. How can you achieve Database Testing using Selenium?
Selenium does not support Database Testing, still, it can be partially done using JDBC and ODBC.
30. How can you prepare a customised HTML report in TestNG using Hybrid framework?
•JUnit with the help of an Ant.
•TestNG using an inbuilt default file.
•Also use an XSL file.