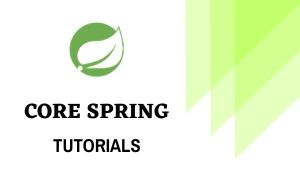
Spring is the most famous application development framework for enterprise Java. Millions of developers around the world use Spring Framework to create high performing, easily testable, and reusable code. Spring framework is an open-source Java platform. It was initially written by Rod Johnson and used to be first released under the Apache two license in June 2003.
Spring is light-weight when it comes to dimension and transparency. The fundamental version of the Spring framework is around 2MB. The core aspects of the Spring Framework can be used in developing any Java application, however, there are extensions for building web applications on top of the Java EE platform. Spring framework objectives to make J2EE development easier to use and promotes good programming practices by enabling a POJO-based programming model.
TOPICS COVERED
FEATURES OF CORE
ARCHITECTURE
DEPENDENCY INJECTION
IOC CONTAINER
SPRING BEAN
ASPECT ORIENTED PROGRAMMING
FEATURES
- Lightweight
Spring is light-weight when it comes to size and transparency. The basic version of the spring framework is around 1MB. And the processing overhead is also very negligible.
- Inversion of control (IOC)
The primary idea of the Dependency Injection or Inversion of Control is that programmer does not want to create the objects, rather just describe how it should be created. No need to directly connect your components and services together in the program, instead just describe which services are needed through which components in a configuration file/XML file. The Spring IOC container is then responsible for binding it all up.
- Aspect-oriented (AOP)
Spring helps Aspect-oriented programming. Aspect-oriented programming refers to the programming paradigm which isolates secondary or supporting functions from the fundamental program’s business logic. AOP is a promising technology for separating cross-cutting concerns, something generally hard to do in object-oriented programming. The application’s modularity is increased in that way and its maintenance turns into significantly easier.
- Container
Spring includes and manages the life cycle and configuration of application objects.
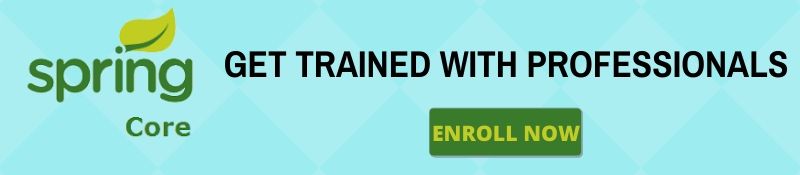
- MVC Framework
Spring comes with an MVC web application framework, built on core Spring functionality. This framework is enormously configurable through method interfaces and contains multiple view technologies like JSP, Velocity, Tiles, iText, and POI. But other frameworks can be easily used as an alternative of the Spring MVC Framework.
- Transaction Management
Spring framework provides a common abstraction layer for transaction management. This allowing the developer to add the pluggable transaction managers, and making it convenient to demarcate transactions besides dealing with low-level issues. Spring’s transaction support is not tied to J2EE environments and it can be additionally used in container much fewer environments.
- JDBC Exception Handling
The JDBC abstraction layer of the Spring gives a significant exception hierarchy, which simplifies the error handling strategy. Integration with Hibernate, JDO, and iBATIS: Spring gives best Integration services with Hibernate, JDO, and iBATIS
ARCHITECTURE
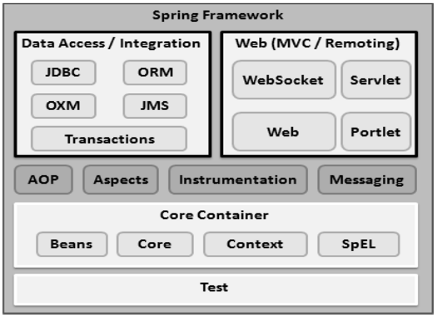
Core Container
The Core Container consists of the Core, Beans, Context, and Expression Language modules the important points of which are as follows :
The Core module gives the essential parts of the framework, consisting of the IoC and Dependency Injection features. The Bean module provides BeanFactory, which is a sophisticated implementation of the factory pattern.
The Context module builds on the strong base provided through the Core and Beans modules and it is a medium to access any objects defined and configured. The ApplicationContext interface is the focal point of the Context module. The SpEL module gives a powerful expression language for querying and manipulating an object graph at runtime.
Data Access/Integration
The Data Access/Integration layer consists of the JDBC, ORM, OXM, JMS and Transaction modules whose element is as follows −
The JDBC module gives a JDBC-abstraction layer that eliminates the need for tedious JDBC related coding.
The ORM module gives integration layers for popular object-relational mapping APIs, consisting of JPA, JDO, Hibernate, and iBatis.
The OXM module offers an abstraction layer that supports Object/XML mapping implementations for JAXB, Castor, XMLBeans, JiBX, and XStream. The Java Messaging Service JMS module consists of features for producing and consuming messages. The Transaction module supports programmatic and declarative transaction management for classes that enforce special interfaces and for all your POJOs.
Web
The Web layer consists of the Web, Web-MVC, Web-Socket, and Web-Portlet modules the details of which are as follows −
The Web module provides primary web-oriented integration features such as multipart file-upload performance and the initialization of the IoC container using servlet listeners and a web-oriented application context. The Web-MVC module consists of Spring’s Model-View-Controller (MVC) implementation for web applications.
The Web-Socket module provides support for WebSocket-based, two-way communication between the client and the server in internet applications. The Web-Portlet module provides the MVC implementation to be used in a portlet environment and mirrors the functionality of the Web-Servlet module.
Miscellaneous
There are few other essential modules like AOP, Aspects, Instrumentation, Web and Test modules the important points of which are as follows −
The AOP module provides an aspect-oriented programming implementation allowing you to outline method-interceptors and pointcuts to cleanly decouple code that implements functionality that has to be separated. The Aspects module gives integration with AspectJ, which is again a powerful and mature AOP framework. The Instrumentation module provides class instrumentation support and classloader implementations to be used in certain application servers. The Messaging module provides support for STOMP as the WebSocket sub-protocol to use in applications. It additionally helps an annotation programming model for routing and processing STOMP messages from WebSocket clients. The Test module supports the testing of Spring components with JUnit or TestNG frameworks.
DEPENDENCY INJECTION (DI)
Dependency injection (DI) is a method whereby objects outline their dependencies, that is, the different objects they work with, only via constructor arguments, arguments to a factory method, or properties that are set on the object instance after it is built or returned from a factory method. The container then injects these dependencies when it creates the bean. This method is essentially the inverse, as a result, the name Inversion of Control (IoC), of the bean itself controlling the instantiation or location of its dependencies on its own through the use of direct construction of classes, or the Service Locator pattern.
The Spring Framework Inversion of Control (IoC) component is the nucleus of the framework. It uses dependency injection to assemble Spring-provided (also known as infrastructure components) and development-provided components in order to rapidly wrap up an application.
Advantages of Dependency Injection
- Decoupling: Code is cleaner with the DI principle and decoupling is more effective when objects are provided with their dependencies.
- Easier to test: As such, your classes become simpler to test, in particular when the dependencies are on interfaces or abstract base classes, which allow for stub or mock implementations to be used in unit tests.
SPRING IOC CONTAINER
Spring IoC is the mechanism to obtain loose-coupling between Object dependencies. To attain free coupling and dynamic binding of the objects at runtime, objects dependencies are injected by other assembler objects. Spring IoC container is the program that injects dependencies into an object and makes it equipped for our use. We have already looked at how we can use Spring Dependency Injection to implement IoC in our applications.
Spring IoC container classes are part of org.springframework.beans, and org.springframework.context packages. Spring IoC container provides us distinctive ways to decouple the object dependencies. Bean Factory is the root interface of the Spring IoC container. Application Context is the child interface of the BeanFactory interface that provides Spring AOP features, i18n, etc. Some of the beneficial child-interfaces of ApplicationContext are ConfigurableApplicationContext and WebApplicationContext. Spring Framework provides a number of useful ApplicationContext implementation training that we can use to get the spring context and then the Spring Bean.
Some of the useful ApplicationContext implementations that we use are:
AnnotationConfigApplicationContext: If we are using Spring in standalone java applications and using annotations for Configuration, then we can use this to initialize the container and get the bean objects.
ClassPathXmlApplicationContext: If we have a spring bean configuration XML file in a standalone application, then we can use this classification to load the file and get the container object.
FileSystemXmlApplicationContext: This is similar to ClassPathXmlApplicationContext except that the xml configuration file can be loaded from anywhere in the file system. AnnotationConfigWebApplicationContext and XmlWebApplicationContext for web applications.
Usually, if you are working on Spring MVC application and your application is configured to use Spring Framework, Spring IoC container gets initialized when the application begins and when a bean is requested, the dependencies are injected automatically. However, for a standalone application, you want to initialize the container somewhere in the application and then use it to get the spring beans.
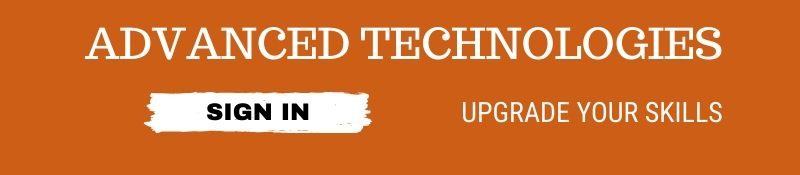
SPRING BEAN
Spring Bean is nothing special, any object in the Spring framework that we initialize through Spring container is known as Spring Bean. Any regular Java POJO class can be a Spring Bean if it’s configured to be initialized by container through providing configuration metadata information.
Spring Bean Scopes
There are 5 scopes defined for Spring Beans.
- Singleton: Only one instance of the bean will be created for each container. This is the default scope for the spring beans. While using this scope, make sure bean doesn’t have shared instance variables in any other case it may lead to data inconsistency issues.
- Prototype: A new instance will be created every time the bean is requested.
- Request: This is same as prototype scope, however, it’s meant to be used for web applications. A new instance of the bean will be created for each HTTP request.
- Session: A new bean will be created for each HTTP session through the container.
- Global-session: This is used to create global session beans for Portlet applications Spring Framework is extendable and we can create our own scopes too. However, most of the time we are accurate with the scopes provided by the framework.
ASPECT ORIENTED PROGRAMMING CORE CONCEPTS
- Aspect: An aspect is a type that implements organization application concerns that reduce across multiple classes, such as transaction management. Aspects can be a regular classification configured via Spring XML configuration or we can use Spring AspectJ integration to outline a category as Aspect using @Aspect annotation.
- Join Point: A join point is a particular point in the application such as method execution, exception handling, altering object variable values, etc. In Spring AOP a join point is usually the execution of a method.
- Advice: Advices are actions taken for a specific join point. In terms of programming, they are techniques that get carried out when a certain join point with a matching pointcut is reached in the application. You can think of Advices as Struts2 interceptors or Servlet Filters.
- Pointcut: Pointcut is expressions that are matched with join points to decide whether advice wants to be performed or not. Pointcut uses different types of expressions that are matched with the join points and the Spring framework uses the AspectJ pointcut expression language.
- Target Object: They are the object on which devices are applied. Spring AOP is implemented using runtime proxies so this object is always a proxied object.
- AOP proxy: Spring AOP implementation makes use of JDK dynamic proxy to create the Proxy classes with target classes and advice invocations, these are referred to as AOP proxy classes. We can additionally use CGLIB proxy by including it as the dependency in the Spring AOP project.
- Weaving: It is the system of linking elements with other objects to create the advised proxy objects. This can be carried out at compile-time, load time, or at runtime. Spring AOP performs weaving at the runtime.