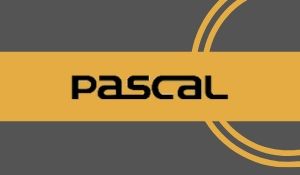
Pascal is a procedural programming language, designed in 1968 and published in 1970 by Niklaus Wirth and named in honour of the French mathematician and philosopher Blaise Pascal. Pascal runs on a range of platforms, such as Windows, Mac OS, and a number of versions of UNIX/Linux. All the features (inheritance, polymorphism, constructors, overloading, pointers, arrays, objects, variables, data types etc..) of pascal will be available in other languages also such as c, java etc.Object Pascal is still utilized for creating Windows based applications additionally can assemble a similar code to iOS and Android etc.
BRIEF ABOUT:
FEATURES OF PASCAL
COMPILERS AND INTERPRETERS
PASCAL DECISION MAKING
FUNCTIONS
ARRAYS
OBJECT ORIENTED PASCAL
FEATURES OF THE PASCAL LANGUAGE
Pascal has the following elements :
- Pascal is a strongly typed language.
- It provides extensive error checking.
- It offers a number of data types like
arrays, records, files and sets. - It gives a range of programming
structures. - It supports structured programming
through functions and procedures. - It supports object oriented programming.
PASCAL COMPILERS AND INTERPRETERS
- Turbo Pascal: gives an IDE and compiler for running Pascal programs on CP/M, CP/M-86, DOS, Windows and Macintosh.
- Delphi: provides compilers for running Object Pascal and generates native cod for 32- and 64-bit Windows operating systems, as well as 32-bit Mac OS X and iOS. Embarcadero is planning to build support for the Linux and Android operating system.
- Free Pascal: it is a free compiler for running Pascal and Object Pascal programs Free Pascal compiler is a 32 and 64 bit Turbo Pascal and Delphi compatible Pascal compiler for Linux, Windows, OS/2, FreeBSD, Mac OS X, DOS and quite a few other platforms.
- Turbo51: it is a free Pascal compiler for the 8051 family of microcontrollers, with Turbo Pascal 7 syntax.
- Oxygene: it is an Object Pascal compiler for the .NET and Mono platforms.
- GNU Pascal (GPC): it is a Pascal compiler composed of a frontend to GNU Compiler Collection.
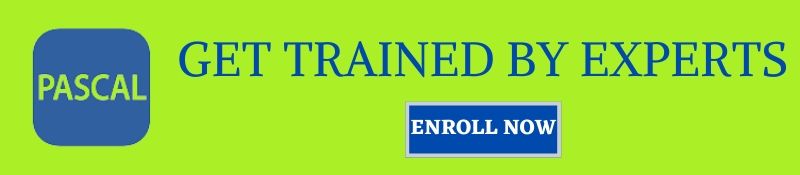
Pascal Statements
Pascal programs are made of statements. Each declaration specifies a precise job of the program. These jobs could be declaration, assignment, reading data, writing data, taking logical decisions, transferring program flow control, etc.
For
example :
readln
(a, b, c);
s
:= (a + b + c)/2.0;
area
:= sqrt(s * (s – a)*(s-b)*(s-c));
writeln(area);
DECISION
MAKING
Decision making structures require that the programmer specify one or more conditions to be evaluated or examined through the program, along with a declaration or statements to be done if the condition is decided to be true, and optionally, other statements to be executed if the condition is determined to be false.
Pascal programming language offers the following kinds of decision making statements.
- if – then statement
An if – then statement consists of a boolean expression followed by one or more statements.
- If-then-else statement
An if – then statement can be followed by using an optional else statement, which executes when the boolean expression is false.
- nested if statements
You
can use one if or else if statement inside any other if or else if statement
- case statement
A
case statement permits a variable to be tested for equality against a list of values.
- case – else statement
It
is similar to the if-then-else statement. Here, an else term follows the case statement.
- nested case statements
You
can use one case statement inside any other case statement.
FUNCTIONS
Subprograms
A
subprogram is a program unit/module that performs a specific task. These subprograms
are mixed to form larger programs. This is basically known as the ‘Modular
design.’ A subprogram can be invoked through a subprogram/program, which is called
the calling program.
Pascal
gives two sorts of subprograms –
Functions
− these subprograms return a single value.
Procedures
− these subprograms do not return a value directly.
Functions
A function is a group of statements that collectively perform a task. Every Pascal program has at least one function, which is the program itself, and all the most trivial programs can outline additional functions.
A function declaration tells the compiler about a function’s name, return type, and parameters. A function definition gives the actual body of the function. Pascal standard library offers numerous built-in functions that your program can call. For example, function AppendStr() appends two strings, function New() dynamically allocates memory to variables and many more functions.
In Pascal, a function is defined using the function keyword. The general form of a function definition is as follows –
function
name(argument(s): type1; argument(s): type2; …): function_type;
local
declarations;
begin
…
< statements >
…
name:= expression;
end;
The
components of a function:
Arguments : The argument(s) set up the linkage between the calling program and the function identifiers and also known as the formal parameters. A parameter is like a placeholder. When a function is invoked, you pass a value to the parameter. This value is referred to as actual parameter or argument. The parameter list refers to the type, order, and number of parameters of a function. Use of such formal parameters is optional. These parameters might also have standard data type, user-defined data type or subrange data type.
The formal parameters list performing in the function statement could be easy or subscripted variables, arrays or structured variables, or subprograms.
Return Type : All functions should return a value, so all functions have to be assigned a type. The function-type is the data type of the value the function returns. It can also be standard, user-defined scalar or subrange type however it can’t be structured type.
Local declarations : Local declarations refer to the declarations for labels, constants, variables, functions and procedures, which are application to the body of function only.
Function Body :The function body consists of a collection of statements that define what the function does. It must constantly be enclosed between the reserved words begin and end. It is the phase of a function where all computations are done. There should be an assignment statement of the type – name := expression; in the function body that assigns a value to the function name. This value is returned as and when the function is executed. The final statement in the body must be an end statement.
PASCAL ARRAYS
Pascal programming language gives a data structure known as the array, which can store a fixed-size sequential series of elements of the same type. An array is used to store a collection of data, however it is frequently more useful to think of an array as a collection of variables of the same type.
Instead of declaring individual variables, such as number1, number2, …, and number100, you declare one array variable such as numbers and use numbers[1], numbers[2], and …, numbers[100] to symbolize character variables. A unique element in an array is accessed through an index.All arrays consist of contiguous memory locations. The lowest address corresponds to the first element and the highest address to the final element.
Please note that if you prefer a C style array beginning from index 0, you just need to begin the index from 0, instead of 1.
Declaring Arrays
To declare an array in Pascal, a programmer can also both declare the kind and then create variables of that array or at once declare the array variable. The common form of type declaration of one-dimensional array is :
type
array-identifier = array[index-type] of element-type;
Where,
array-identifier − shows the name of the array type.
index-type − specifies the subscript of the array; it can be any scalar data type except real
element-type − specifies the types of values that are going to be stored
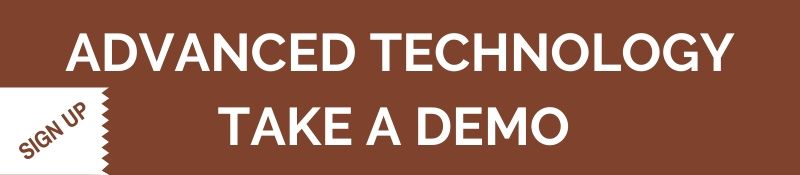
OBJECT-ORIENTED PASCAL.
Object: An Object is a unique type of record that consists of fields like a record; however, unlike records, objects include procedures and functions as part of the object. These procedures and functions are held as pointers to the methods related with the object’s type.
Class: A Class is defined in almost the same way as an Object, however there is a difference in way they are created. The Class is allotted on the Heap of a program, whereas the Object is allocated on the Stack. It is a pointer to the object, not the object itself.
Instantiation of a class: Instantiation means developing a variable of that class type. Since a class is just a pointer, when a variable of a class type is declared, there is memory allocated only for the pointer, not for the whole object. Only when it is instantiated using one of its constructors, memory is allocated for the object. Instances of a class are also known as ‘objects’, but do not confuse them with Object Pascal Objects.
Member Variables: These are the variables described inside a Class or an Object.
Member Functions: These are the functions or methods defined inside a Class or an Object and are used to access object data.
Visibility of Members: The members of an Object or Class are also known as the fields. These fields have unique visibilities. Visibility refers to accessibility of the members, i.e., exactly where these members will be accessible. Objects have three visibility levels: public, private and protected.
Classes have 5 visibility types: public, private, strictly private, protected and published.
Inheritance: When a Class is described by inheriting existing functionalities of a parent Class, then it is stated to be inherited. Here child class will inherit all or few member functions and variables of a parent class. Objects can additionally be inherited.
Parent Class: A Class that is inherited through another Class . This is also called a base class or super class.
Child Class: A class that inherits from another class. This is also referred to as a subclass or derived class.
Polymorphism: This is an object-oriented concept where same function can be used for unique purposes. For example, function name will remain same however it may take unique number of arguments and can do different tasks. Pascal classes implement polymorphism. Objects do not implement polymorphism.
Overloading: It is a kind of polymorphism in which some or all of operators have different implementations relying on the types of their arguments. Similarly functions can additionally be overloaded with different implementation. Pascal classes implement overloading, however the Objects do not.
Data Abstraction: Any representation of data in which the implementation details are hidden (abstracted).
Encapsulation: Refers to a concept where we encapsulate all the data and member functions together to form an object.
Constructor: Refers to a different kind of function which will be called automatically whenever there is an object formation from a class or an Object.
Destructor: Refers to a unique kind of function which will be called automatically each time an Object or Class is deleted or goes out of scope.
The general form of an object declaration:
type object-identifier = object
private
field1 : field-type;
field2 : field-type;
…
public
procedure proc1;
function f1(): function-type;
end;
var objectvar : object-identifier;